???????????????????ó???
???????????? ???????[ 2016/8/3 16:57:56 ] ??????????????? ???????
??д???????
[Specification]
public void InsertPage_should_insert_a_page_in_database_and_cache_it()
{
var cache = new Mock<ICache>();
var database = new Mock<IDropthingsDataContext>();
IPageRepository pageRepository = new PageRepository(database.Object?? cache.Object);
const int pageId = 1;
var page = default(Page);
var samplePage = new Page() { ID = pageId?? Title = "Test Page"?? ColumnCount = 3??
LayoutType = 3?? UserId = Guid.NewGuid()?? VersionNo = 1??
PageType = Enumerations.PageTypeEnum.PersonalPage?? CreatedDate = DateTime.Now };
database
.Expect<Page>(d => d.Insert<Page>(DropthingsDataContext.SubsystemEnum.Page??
It.IsAny<Action<Page>>()))
.Returns(samplePage);
"Given PageRepository".Context(() =>
{
// It will clear items from cache
cache.Expect(c => c.Remove(CacheSetup.CacheKeys.PagesOfUser(samplePage.UserId)));
});
"when Insert is called".Do(() =>
page = pageRepository.Insert((newPage) =>
{
newPage.Title = samplePage.Title;
newPage.ColumnCount = samplePage.ColumnCount;
newPage.LayoutType = samplePage.LayoutType;
newPage.UserId = samplePage.UserId;
newPage.VersionNo = samplePage.VersionNo;
newPage.PageType = samplePage.PageType;
}));
("then it should insert the page in database" +
"and clear any cached collection of pages for the user who gets the new page" +
"and it returns the newly inserted page").Assert(() =>
{
database.VerifyAll();
cache.VerifyAll();
Assert.Equal<int>(pageId?? page.ID);
});
}
??????????д?Щ???????PageRepository.Insert??????????????μ?Page????????fail??????????????????????????????????????????????????????????????
public Page Insert(Action<Page> populate)
{
return new Page();
}
???й??????????????
TestCase ‘Given PageRepository when InsertPage is called?? then it should insert the
page in databaseand clear any cached collection of pages for the user who gets the
new pageand it returns the newly inserted page’
failed: Moq.MockVerificationException : The following expectations were not met:
IDropthingsDataContext d => d.Insert(Page?? null)
at Moq.Mock`1.VerifyAll()
PageRepositoryTest.cs(278??0): at
Dropthings.DataAccess.UnitTest.PageRepositoryTest.<>c__DisplayClass35.
b__34()
?????????к??database.Insert ??????????????????????TDD????????????д???????????????????????????????????????顣
????????????????
public Page Insert(Action<Page> populate)
{
var newPage = _database.Insert<Page>(
DropthingsDataContext.SubsystemEnum.Page?? populate);
RemoveUserPagesCollection(newPage.UserId);
return newPage.Detach();
}
??????????????????PageRepository ????????μ???????????????????
???BDD???м?????????????????
???????????????????TDD???????????д??????????д??????????????????????? ????????Web????TDD?????????????????????д?????????????????????????????л????????????????????????????????????????????????????????????????????????????????????????????????????????磬?????FirstVisitHomePage ????????????????η????????????д??????????檔????????????鷵?????????????????????????????????η??????????????????????????????????????????????????????????????
????????:
public void Revisit_should_load_the_pages_and_widgets_exactly_the_same()
{
MembershipHelper.UsingNewAnonUser((profile) =>
{
using (var facade = new Facade(new AppContext(string.Empty?? profile.UserName)))
{
UserSetup userVisitModel = null;
UserSetup userRevisitModel = null;
"Given an anonymous user who visited first".Context(() =>
{
userVisitModel = facade.FirstVisitHomePage(profile.UserName?? ...);
});
"when the same user visits again".Do(() =>
{
userRevisitModel = facade.RepeatVisitHomePage(profile.UserName?? ...);
});
"it should load the exact same pages?? column and
widgets as the first visit produced".Assert(() =>
{
userVisitModel.UserPages.Each(firstVisitPage =>
{
Assert.True(userRevisitModel.UserPages.Exists(page =>
page.ID == firstVisitPage.ID));
var revisitPage = userRevisitModel.UserPages.First(page =>
page.ID == firstVisitPage.ID);
var revisitPageColumns = facade.GetColumnsInPage(revisitPage.ID);
facade.GetColumnsInPage(firstVisitPage.ID).Each(firstVisitColumn =>
{
var revisitColumn = revisitPageColumns.First(column =>
column.ID == firstVisitColumn.ID);
var firstVisitWidgets = facade
.GetWidgetInstancesInZoneWithWidget(firstVisitColumn.WidgetZoneId);
var revisitWidgets = facade
.GetWidgetInstancesInZoneWithWidget(revisitColumn.WidgetZoneId);
firstVisitWidgets.Each(firstVisitWidget =>
Assert.True(revisitWidgets.Where(revisitWidget =>
revisitWidget.Id == firstVisitWidget.Id).Count() == 1));
});
});
});
}
});
}
????????????????????д????????????檔?????????У??????????????????????????????????????????ò????????????????????????????????????????????????????????????????????????????????????????????
* ???????????????????????磬????????в????л????Web???????????????壩???????????????????У?
??????????ζ?????л?????????Web?????????????????????????????????????б???????????磬???????У???????? ?????????????????
?????????????????????β??????л???????Υ??????????????????????????????????????顣
* ???????????????????????磬??????????е??У????????????????????????????
?????????o???????????????????????????????????????????????????????????????????????????????????
?????????3?????????????????????????糢???????o????????????????????в??????С????????????????顣
* ???????????Щ?????????????????????????
?????????????????????2??????в??????Υ??????????????????????
??У????????????????????????????????????????У??????????????
??????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????????澡?????????????????????????
??????
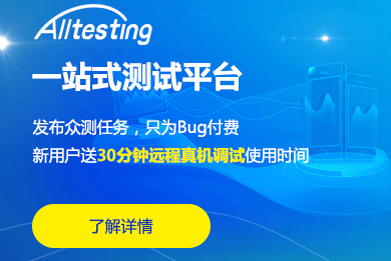
???·???
??????????????????
2023/3/23 14:23:39???д?ò??????????
2023/3/22 16:17:39????????????????????Щ??
2022/6/14 16:14:27??????????????????????????
2021/10/18 15:37:44???????????????
2021/9/17 15:19:29???·???????·
2021/9/14 15:42:25?????????????
2021/5/28 17:25:47??????APP??????????
2021/5/8 17:01:11